Maintainers
This module is maintained by the OCA.
-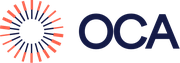
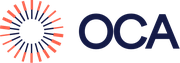
OCA, or the Odoo Community Association, is a nonprofit organization whose mission is to support the collaborative development of Odoo features and promote its widespread use.
diff --git a/fastapi/tests/test_fastapi.py b/fastapi/tests/test_fastapi.py index f3b22aad..dcc7385b 100644 --- a/fastapi/tests/test_fastapi.py +++ b/fastapi/tests/test_fastapi.py @@ -3,8 +3,15 @@ import os import unittest +from contextlib import contextmanager +from odoo import sql_db from odoo.tests.common import HttpCase +from odoo.tools import mute_logger + +from fastapi import status + +from ..schemas import DemoExceptionType @unittest.skipIf(os.getenv("SKIP_HTTP_CASE"), "EndpointHttpCase skipped") @@ -21,6 +28,13 @@ def setUpClass(cls): ) lang.active = True + @contextmanager + def _mocked_commit(self): + with unittest.mock.patch.object( + sql_db.TestCursor, "commit", return_value=None + ) as mocked_commit: + yield mocked_commit + def _assert_expected_lang(self, accept_language, expected_lang): route = "/fastapi_demo/demo/lang" response = self.url_open(route, headers={"Accept-language": accept_language}) @@ -38,3 +52,108 @@ def test_lang(self): self._assert_expected_lang("en,fr;q=0.7,en-GB;q=0.3", b'"en_US"') self._assert_expected_lang("fr-FR,en;q=0.7,en-GB;q=0.3", b'"fr_BE"') self._assert_expected_lang("fr-FR;q=0.1,en;q=1.0,en-GB;q=0.8", b'"en_US"') + + def test_retrying(self): + """Test that the retrying mechanism is working as expected with the + FastAPI endpoints. + """ + nbr_retries = 3 + route = f"/fastapi_demo/demo/retrying?nbr_retries={nbr_retries}" + response = self.url_open(route, timeout=20) + self.assertEqual(response.status_code, 200) + self.assertEqual(int(response.content), nbr_retries) + + @mute_logger("odoo.http") + def assert_exception_processed( + self, + exception_type: DemoExceptionType, + error_message: str, + expected_message: str, + expected_status_code: int, + ) -> None: + with self._mocked_commit() as mocked_commit: + route = ( + "/fastapi_demo/demo/exception?" + f"exception_type={exception_type.value}&error_message={error_message}" + ) + response = self.url_open(route, timeout=200) + mocked_commit.assert_not_called() + self.assertDictEqual( + response.json(), + { + "detail": expected_message, + }, + ) + self.assertEqual(response.status_code, expected_status_code) + + def test_user_error(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.user_error, + error_message="test", + expected_message="test", + expected_status_code=status.HTTP_400_BAD_REQUEST, + ) + + def test_validation_error(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.validation_error, + error_message="test", + expected_message="test", + expected_status_code=status.HTTP_400_BAD_REQUEST, + ) + + def test_bare_exception(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.bare_exception, + error_message="test", + expected_message="Internal Server Error", + expected_status_code=status.HTTP_500_INTERNAL_SERVER_ERROR, + ) + + def test_access_error(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.access_error, + error_message="test", + expected_message="AccessError", + expected_status_code=status.HTTP_403_FORBIDDEN, + ) + + def test_missing_error(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.missing_error, + error_message="test", + expected_message="MissingError", + expected_status_code=status.HTTP_404_NOT_FOUND, + ) + + def test_http_exception(self) -> None: + self.assert_exception_processed( + exception_type=DemoExceptionType.http_exception, + error_message="test", + expected_message="test", + expected_status_code=status.HTTP_409_CONFLICT, + ) + + @mute_logger("odoo.http") + def test_request_validation_error(self) -> None: + with self._mocked_commit() as mocked_commit: + route = "/fastapi_demo/demo/exception?exception_type=BAD&error_message=" + response = self.url_open(route, timeout=200) + mocked_commit.assert_not_called() + self.assertEqual(response.status_code, status.HTTP_422_UNPROCESSABLE_ENTITY) + + def test_no_commit_on_exception(self) -> None: + # this test check that the way we mock the cursor is working as expected + # and that the transaction is rolled back in case of exception. + with self._mocked_commit() as mocked_commit: + url = "/fastapi_demo/demo" + response = self.url_open(url, timeout=600) + self.assertEqual(response.status_code, 200) + mocked_commit.assert_called_once() + + self.assert_exception_processed( + exception_type=DemoExceptionType.http_exception, + error_message="test", + expected_message="test", + expected_status_code=status.HTTP_409_CONFLICT, + ) diff --git a/fastapi/tests/test_fastapi_demo.py b/fastapi/tests/test_fastapi_demo.py index 5cd9fef5..1692e69f 100644 --- a/fastapi/tests/test_fastapi_demo.py +++ b/fastapi/tests/test_fastapi_demo.py @@ -2,17 +2,14 @@ # License LGPL-3.0 or later (http://www.gnu.org/licenses/LGPL). from functools import partial -from unittest import mock from requests import Response -from odoo.tools import mute_logger - from fastapi import status from ..dependencies import fastapi_endpoint from ..routers import demo_router -from ..schemas import DemoEndpointAppInfo, DemoExceptionType +from ..schemas import DemoEndpointAppInfo from .common import FastAPITransactionCase @@ -34,36 +31,6 @@ def setUpClass(cls) -> None: {"name": "FastAPI Demo"} ) - @mute_logger("odoo.addons.fastapi.error_handlers") - def assert_exception_processed( - self, - exception_type: DemoExceptionType, - error_message: str, - expected_message: str, - expected_status_code: int, - ) -> None: - demo_app = self.env.ref("fastapi.fastapi_endpoint_demo") - with self._create_test_client( - demo_app._get_app(), raise_server_exceptions=False - ) as test_client, mock.patch.object( - self.env.cr.__class__, "rollback" - ) as mock_rollback: - response: Response = test_client.get( - "/demo/exception", - params={ - "exception_type": exception_type.value, - "error_message": error_message, - }, - ) - mock_rollback.assert_called_once() - self.assertEqual(response.status_code, expected_status_code) - self.assertDictEqual( - response.json(), - { - "detail": expected_message, - }, - ) - def test_hello_world(self) -> None: with self._create_test_client() as test_client: response: Response = test_client.get("/demo/") @@ -94,51 +61,3 @@ def test_endpoint_info(self) -> None: response.json(), DemoEndpointAppInfo.model_validate(demo_app).model_dump(by_alias=True), ) - - def test_user_error(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.user_error, - error_message="test", - expected_message="test", - expected_status_code=status.HTTP_400_BAD_REQUEST, - ) - - def test_validation_error(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.validation_error, - error_message="test", - expected_message="test", - expected_status_code=status.HTTP_400_BAD_REQUEST, - ) - - def test_bare_exception(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.bare_exception, - error_message="test", - expected_message="test", - expected_status_code=status.HTTP_500_INTERNAL_SERVER_ERROR, - ) - - def test_access_error(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.access_error, - error_message="test", - expected_message="AccessError", - expected_status_code=status.HTTP_403_FORBIDDEN, - ) - - def test_missing_error(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.missing_error, - error_message="test", - expected_message="MissingError", - expected_status_code=status.HTTP_404_NOT_FOUND, - ) - - def test_http_exception(self) -> None: - self.assert_exception_processed( - exception_type=DemoExceptionType.http_exception, - error_message="test", - expected_message="test", - expected_status_code=status.HTTP_409_CONFLICT, - )